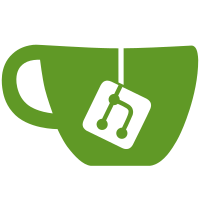
* Add ServerRegistration, begin refactoring to match frontend * move graphData logic into ServerRegistration * move ping updates/history into ServerRegistration * start updating main app entry methods * fix default rates.updateMojangStatus * fix record loading delays on freshly booted instances * move database loading logic to method + callback * use data in frontend for type lookup instead of ping * cleanup app.js * reorganize methods to improve flow * avoid useless mojang updates, remove legacy fields * rename legacy fields for consistency * finish restructure around App model * ensure versions are sorted by release order * filter errors sent to frontend to avoid data leaks * fix version listing behavior on frontend * 5.1.0
27 lines
968 B
JavaScript
27 lines
968 B
JavaScript
const MOJANG_STATUS_BASE_CLASS = 'header-button header-button-group'
|
|
|
|
export class MojangUpdater {
|
|
updateStatus (services) {
|
|
for (const name of Object.keys(services)) {
|
|
this.updateServiceStatus(name, services[name])
|
|
}
|
|
}
|
|
|
|
updateServiceStatus (name, title) {
|
|
// HACK: ensure mojang-status is added for alignment, replace existing class to swap status color
|
|
document.getElementById('mojang-status_' + name).setAttribute('class', MOJANG_STATUS_BASE_CLASS + ' mojang-status-' + title.toLowerCase())
|
|
document.getElementById('mojang-status-text_' + name).innerText = title
|
|
}
|
|
|
|
reset () {
|
|
// Strip any mojang-status-* color classes from all mojang-status classes
|
|
document.querySelectorAll('.mojang-status').forEach(function (element) {
|
|
element.setAttribute('class', MOJANG_STATUS_BASE_CLASS)
|
|
})
|
|
|
|
document.querySelectorAll('.mojang-status-text').forEach(function (element) {
|
|
element.innerText = '...'
|
|
})
|
|
}
|
|
}
|